Being a developer requires continuous problem solving and constant growth. This is especially true when it comes to iOS/Swift development as Swift is changing very dynamically and Apple constantly comes up with new solutions for better UX or quicker apps development. Alongside with language development, the way that developers think about the code changes as well.
They incessantly think about how to improve their code’s quality. They see patterns in their code and universal solutions in their apps. They move them out and create frameworks. At the moment, there is a huge amount of frameworks for Swift/iOS on GitHub that every developer can use. Moreover, not only do developers use those frameworks but also contribute to the creation and development of them. This is caused by the fact that we value our time and we don’t want to spend it on “inventing the wheel” - creating solutions that have been already created by another developer.
Swift is built on the many developers’ contribution and we’re inspired by this example that the community of developers can create greater things as a team than they could as individuals. Those are the reasons why developers even bother with creating open-source frameworks. Some of them were the reasons why our team created Restler.
Table of Contents:
1. What is the framework in iOS Mobile App Development?
2. What is Restler and Why Have We Decided to Build it?
3. Restler iOS framework code examples.
4. 4 Situations When You Should Consider Writing Your Own Framework.
5. 3 Situation When You Should NOT Create Your Own Framework.
What is the framework in iOS Mobile App Development?
But before we start looking at Restler, let’s explain what we mean under the term “framework”. The framework we talk about is some isolated part of a software that can be used by another software to support some functionalities. It sounds very complicated but it’s pretty easy to understand if we have a good example.
Let’s say we have a well-working car. The car needs an engine, pumps, brakes and many other parts to work. Any of these parts works without being in the car and each of them does one thing that it was destined to - brakes stop the wheel, pumps pump coolant or brake liquid, the engine makes wheels spinning and other stuff working. So putting this example into the programming world, the car is an app we create, and the engine, pumps, and breaks are frameworks we use in the app to make it work.
What is Restler and Why Have We Decided to Build it?
On GitHub you can find multiple different frameworks solving problems that developers face when it comes to adding some functionalities or supporting basic app’s functionality. For example, iOS mobile developers use Networking or Alamofire for networking layer, CoreStore as a wrapper of Apple’s CoreData which is very difficult to use by yourself, KeychainAccess for storing sensitive data safely on the user’s device and much more.
Restler framework which we’ve created at Railwaymen can be used in multiple different applications for handling API requests used for communicating with the backend. It’s very universal because nowadays almost every mobile application uses such requests e.g. when you check your Facebook board or send a post on Twitter. It was created because we had noticed a pattern in handling network requests which had been repeated in iOS projects we had been working on. The frameworks that we used, Networking and Alamofire, were stable and fine for our purposes but we wanted to have a different interface to handle code responsible for decoding responses from the backend to our structures - the piece of code we had to repeat in every project. Moreover, we were inspired by Vapor - a framework for writing the server-side with Swift - and we wanted to build an interface that was clear, simple, and descriptive.
We achieved these goals and integrated it into our internal project for counting work time, requesting vacation or remote work, to test its possibilities and limitations. Since we finished it, we have noticed that our app has got smaller - the whole functionality responsible for wrapping the previous framework we used has just disappeared. That means we don’t need to copy-paste the solution in multiple projects, but we can simply import the package and use it.
Restler iOS framework code examples
We wanted Restler to be simple - so you could use it quickly without thinking too much about how URLSession or URLRequest (frameworks provided to us by Apple) work. Restler builds and calls it for you.
At the beginning, after we installed it, we should initialize Restler:
guard let url = URL(string: “https://example.com/api”) else { return }
let restler = Restler(baseURL: url)
The base URL is simply a link to the website where you are going to do your requests.
Next, you can already call it:
let task = restler
.get(“/users”) // 1
.query([“id”: 1]) // 2
.decode(User.self) // 3
.onSuccess { print(“User:”, $0.firstName) } // 4
.start() // 5
- Make GET request to “users” endpoint
- Add “?id=1” query to the url
- On successful request decode User structure
- Print user’s first name on successful request
- Start the built request
It’s so quick and easy, isn’t it?
We could also use multipart encoding type for our POST request:
let task = restler
.post(“/posts”) // 1
.multipart(ourMultipartObject) // 2
.decode(Post?.self) // 3
.onFailure { print(“Request failed with error:”, $0.localizedDescription) } // 4
.start() // 5
- Make POST request to “posts” endpoint
- Encode the object as multipart content
- Try to decode Post object - if decoding failed, nil will be returned
- Print error on failure
- Start the built request
You could ask - OK, but what is “ourMultipartObject”? This is a structure conforming to a protocol named “RestlerMultipartEncodable”. The implementation is very similar to the implementation of the “Encodable” protocol known to developers, so it shouldn't be a problem for them.
The task Restler’s “start” function returns is a task we have started. It is created with the purpose of checking its state or canceling it. This is very helpful when we want to optimize the network requests.
We can actually split the call of Restler into two parts: request building and response handling. Let’s take a look at this example:
let task = restler
// At the beginning, we want to set basic elements of request like method, headers, contained values etc.
.get(“/users”)
.query([“id”: 1])
.setInHeader(“myTempToken”, forKey: “token”)
// Here ends the part of setting up the request
// And begins setting up handling of the response
.failureDecode(MyCustomDecodableError.self)
.decode(User.self)
.onSuccess { print(“User:”, $0.firstName) }
// Finally, we start the request
.start()
If you notice this split in the Restler’s request builder, everything will get a little bit easier to understand like, for example, the order of calls.
It supports all methods - GET, POST, PUT, PATCH, DELETE, and even HEAD. We can encode content of the POST or PUT request simply providing our decodable structure into a “body” function. It is also planned to support other cool functionalities (including Apple’s Combine framework). All documentation you can find on GitHub.
4 Situations When You Should Consider Writing Your Own Framework
You still could wonder why we bothered with creating our own framework for networking requests if there were already existing solutions like Networking or Alamofire which are very popular and always support the latest iOS version. It’s because we needed a simple interface that such big frameworks like these didn’t have.
#1 You need a simpler interface for another framework.
We used the Networking framework, but its interface was still a little bit too complicated. We needed something simple and quick in use. We wanted to do some wrapper on Networking but it didn’t require that much effort to avoid using third-party solutions and use the interface provided by Apple that was not that hard to implement. For example, a framework handling in-app purchases would be much harder to build and it would be more fragile.
#2 You see repeated code in your multiple applications doing exactly the same thing.
We had noticed that we were writing the same algorithm, same error handling and same tests in a few projects and this was time-consuming. And time means money! If you are now thinking that creating a framework is time-consuming - yes it is, but it is worth doing it if you have to repeat the same thing in multiple places. Simply imagine if somewhere in your logic is a bug. You have to dig through all of the apps you’ve made, fix the bug and write tests. If you move the logic out of the app to the framework, you simply fix it in one place and update the dependency in all apps using it. This is much easier to do.
#3 You see a set of classes that should work independently (a kind of an engine).
This point is similar to the previous one but not the same. The interface that we were building in some projects was something independent from the whole application. It would work for any application or any device and it was used only by one class which manages the whole networking layer. Exactly the same rule exists in building car engines and games’ engines. A car engine isn’t welded with the car so much that you cannot split it off. It is one working part which you can unmount, and which can be used by almost any vehicle but it’s just adjusted to being used in the car.
The framework doesn’t have to be universal in this case and it doesn’t have to be open-source. We should separate modules in our app even if they are useful only in this application. This approach improves the testability and readability of our codebase.
#4 The framework you’re about to use is a tank.
No mechanic would build a car with a tank’s engine. Frameworks must be adjusted for usage in our application’s type so the framework isn’t X times heavier (is a size or another resource usage) than it supposed to be. So Restler is lightweight and doesn’t contain functionalities we don’t need. That’s also the reason why we wanted to wrap the interface provided by Apple rather than some third-party library. (Apple’s frameworks are already installed on its systems like iOS so they don’t increase the size of an app)
3 Situation When You Should NOT Create Your Own Framework
But it’s not like building your own framework is the best choice in every situation that occurs during the app development process. I’ll give you some advice when you shouldn’t do it:
#1 The solution for what you want to do already exists and it fits your needs.
If your framework would be just a copy of another already existing framework, this would be just a waste of time. The developers’ time is very valuable and it shouldn’t be spent on things that won’t have an impact on the developer’s skills/knowledge or on the community.
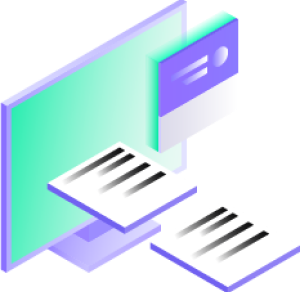
Now we want to share it with you!
#2 The framework doesn’t solve a real problem.
The best frameworks arise in need. Then they are solving a problem that is common in many applications. Or even if it’s not common, it is a real problem which we have to solve in one way or another but we cannot bypass solving it.
#3 You don’t have any idea how to separate your code.
The first step in creating a framework is to have a list of goals we want to achieve and plan how we could achieve them. This step should be done even before creating a repository for our new framework. This is because if we have goals, we can be sure that our framework solves the real problems, not some imaginary ones.
Conclusion
As we could see, to create new frameworks, the developer needs some experience and a will to learn new things. But it is something that every developer should go through because it is one step further in increasing the speed of development and skills of the developer contributing to the framework creation. Moreover, to create a framework, developers have to show their creativity, because they are creating a new solution which provides new possibilities and reduces the size of a codebase which also improves the maintainability of the project.
Faster development means lower costs and skilled developers mean better quality of a product, so it’s better to choose companies that invest in open-source solutions. Interested in what kind of apps have we already made?